TensorFlow.js 教程
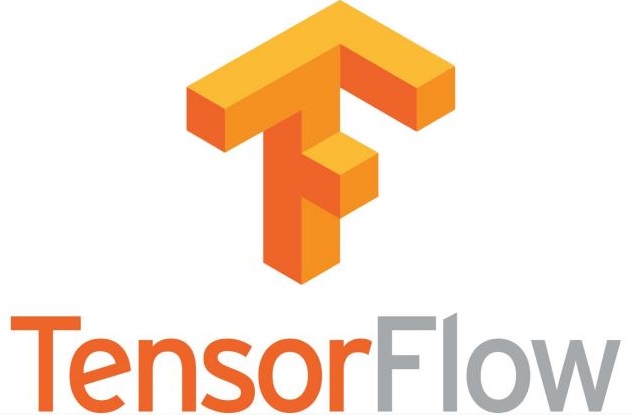
什么是 TensorFlow.js?
Tensorflow 是一个流行的 JavaScript 库,用于 机器学习。
Tensorflow 让我们可以在 浏览器 中训练和部署机器学习。
Tensorflow 让我们可以向任何 Web 应用程序 添加机器学习功能。
使用 TensorFlow
要使用 TensorFlow.js,请将以下脚本标签添加到您的 HTML 文件中
示例
<script src="https://cdn.jsdelivr.net.cn/npm/@tensorflow/[email protected]/dist/tf.min.js"></script>
如果您始终想要使用最新版本,请删除版本号
示例 2
<script src="https://cdn.jsdelivr.net.cn/npm/@tensorflow/tfjs"></script>
TensorFlow 由 Google Brain 团队 开发,最初供 Google 内部使用,但在 2015 年发布为开源软件。
2019 年 1 月,Google 开发人员发布了 TensorFlow.js,它是 TensorFlow 的 JavaScript 实现。
Tensorflow.js 的设计目标是提供与用 Python 编写的原始 TensorFlow 库相同的功能。
张量
TensorFlow.js 是一个 JavaScript 库,用于定义和操作 张量。
TensorFlow.js 中的主要数据类型是 张量。
张量 与多维数组非常相似。
张量 包含一个或多个维度中的值
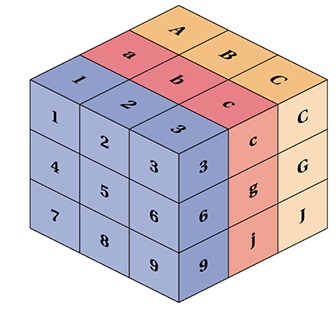
张量 具有以下主要属性
属性 | 描述 |
---|---|
dtype | 数据类型 |
rank | 维数 |
shape | 每个维度的尺寸 |
在机器学习中,术语“维度”有时与“秩”互换使用。
[10, 5] 是一个二维张量或一个二维张量。
此外,术语“维度”可以指一个维度的尺寸。
示例:在二维张量 [10, 5] 中,第一维的维度为 10。
创建张量
TensorFlow 中的主要数据类型是 张量。
使用 tf.tensor() 方法,可以从任何 N 维数组创建张量
示例 1
const myArr = [[1, 2, 3, 4]];
const tensorA = tf.tensor(myArr);
示例 2
const myArr = [[1, 2], [3, 4]];
const tensorA = tf.tensor(myArr);
示例 3
const myArr = [[1, 2], [3, 4], [5, 6]];
const tensorA = tf.tensor(myArr);
张量形状
张量也可以从 数组 和 形状 参数创建
示例 1
const myArr = [1, 2, 3, 4]
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
示例 2
const tensorA = tf.tensor([1, 2, 3, 4], [2, 2]);
示例 3
const myArr = [[1, 2], [3, 4]];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
检索张量值
您可以使用 tensor.data() 获取张量背后的 数据
示例
const myArr = [[1, 2], [3, 4]];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
tensorA.data().then(data => display(data));
function display(data) {
document.getElementById("demo").innerHTML = data;
}
您可以使用 tensor.array() 获取张量背后的 数组
示例
const myArr = [[1, 2], [3, 4]];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
tensorA.array().then(array => display(array[0]));
function display(data) {
document.getElementById("demo").innerHTML = data;
}
const myArr = [[1, 2], [3, 4]];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
tensorA.array().then(array => display(array[1]));
function display(data) {
document.getElementById("demo").innerHTML = data;
}
您可以使用 tensor.rank 获取张量的 秩
示例
const myArr = [1, 2, 3, 4];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
document.getElementById("demo").innerHTML = tensorA.rank;
您可以使用 tensor.shape 获取张量的 形状
示例
const myArr = [1, 2, 3, 4];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
document.getElementById("demo").innerHTML = tensorA.shape;
您可以使用 tensor.dtype 获取张量的 数据类型
示例
const myArr = [1, 2, 3, 4];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape);
document.getElementById("demo").innerHTML = tensorA.dtype;
张量数据类型
张量可以具有以下数据类型
- bool
- int32
- float32(默认)
- complex64
- string
创建张量时,您可以将数据类型指定为第三个参数
示例
const myArr = [1, 2, 3, 4];
const shape = [2, 2];
const tensorA = tf.tensor(myArr, shape, "int32");