操作指南 - 放大镜
学习如何创建一个图像放大镜。
图像放大镜
将鼠标悬停在图像上
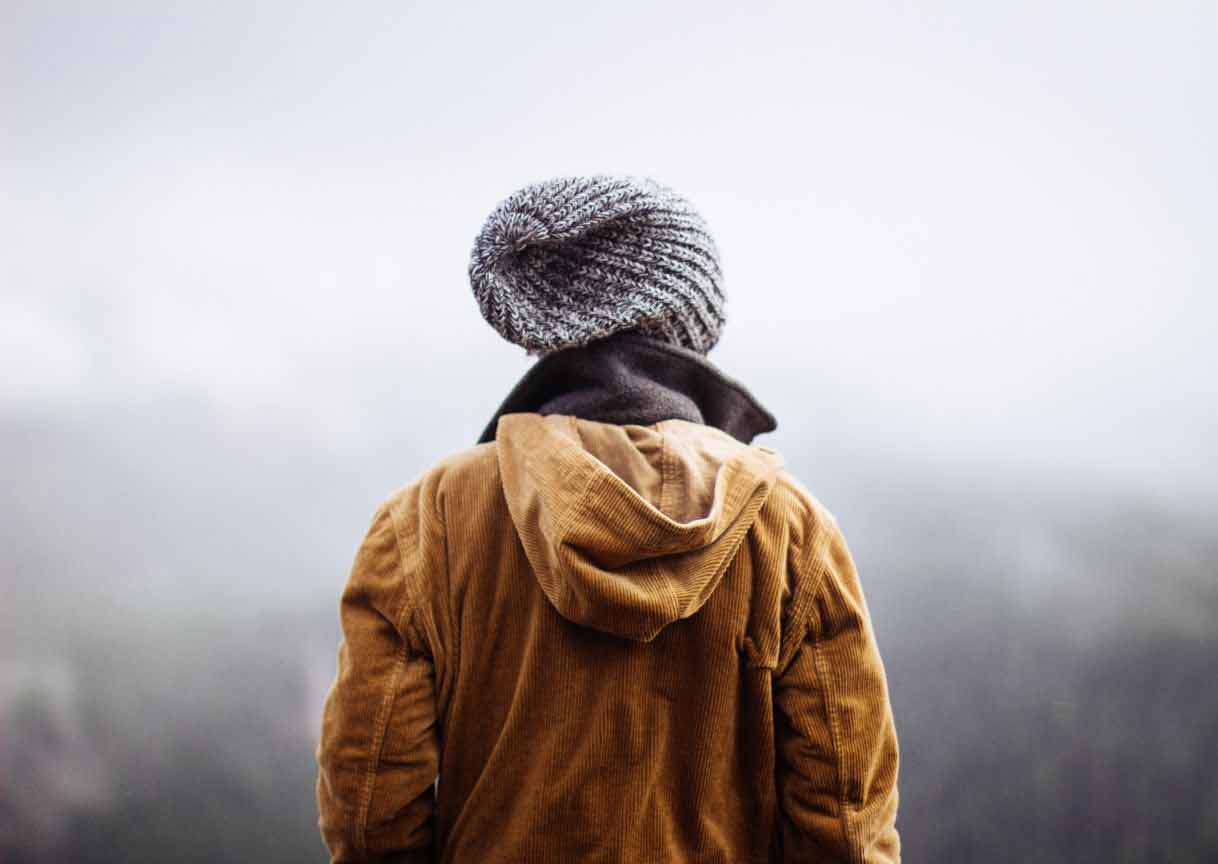
创建一个图像放大镜
步骤 1) 添加 HTML
示例
<div class="img-magnifier-container">
<img id="myimage" src="img_girl.jpg" width="600" height="400" alt="女孩">
</div>
步骤 2) 添加 CSS
容器必须具有“相对”定位。
示例
* {box-sizing: border-box;}
.img-magnifier-container {
position: relative;
}
.img-magnifier-glass {
position: absolute;
border: 3px solid #000;
border-radius: 50%;
cursor: none;
/* 设置放大镜的大小:*/
width: 100px;
height: 100px;
}
步骤 3) 添加 JavaScript
示例
function magnify(imgID, zoom) {
var img, glass, w, h, bw;
img = document.getElementById(imgID);
/* 创建放大镜: */
glass = document.createElement("DIV");
glass.setAttribute("class", "img-magnifier-glass");
/* 插入放大镜: */
img.parentElement.insertBefore(glass, img);
/* 设置放大镜的背景属性: */
glass.style.backgroundImage = "url('" + img.src + "')";
glass.style.backgroundRepeat = "no-repeat";
glass.style.backgroundSize = (img.width * zoom) + "px " + (img.height * zoom) + "px";
bw = 3;
w = glass.offsetWidth / 2;
h = glass.offsetHeight / 2;
/* 当有人将放大镜移过图像时,执行一个函数: */
glass.addEventListener("mousemove", moveMagnifier);
img.addEventListener("mousemove", moveMagnifier);
/* 以及触摸屏:*/
glass.addEventListener("touchmove", moveMagnifier);
img.addEventListener("touchmove", moveMagnifier);
function moveMagnifier(e) {
var pos, x, y;
/* 阻止移动图像时可能发生的任何其他操作 */
e.preventDefault();
/* 获取光标的 x 和 y 位置: */
pos = getCursorPos(e);
x = pos.x;
y = pos.y;
/* 阻止放大镜定位在图像之外: */
if (x > img.width - (w / zoom)) {x = img.width - (w / zoom);}
if (x < w / zoom) {x = w / zoom;}
if (y > img.height - (h / zoom)) {y = img.height - (h / zoom);}
if (y < h / zoom) {y = h / zoom;}
/* 设置放大镜的位置: */
glass.style.left = (x - w) + "px";
glass.style.top = (y - h) + "px";
/* 显示放大镜“看到”的内容: */
glass.style.backgroundPosition = "-" + ((x * zoom) - w + bw) + "px -" + ((y * zoom) - h + bw) + "px";
}
function getCursorPos(e) {
var a, x = 0, y = 0;
e = e || window.event;
/* 获取图像的 x 和 y 位置: */
a = img.getBoundingClientRect();
/* 计算光标的 x 和 y 坐标,相对于图像: */
x = e.pageX - a.left;
y = e.pageY - a.top;
/* 考虑任何页面滚动: */
x = x - window.pageXOffset;
y = y - window.pageYOffset;
return {x : x, y : y};
}
}