Vue 路由
路由 在 Vue 中用于导航 Vue 应用程序,它在客户端(在浏览器中)进行,无需完整页面重新加载,从而带来更快的用户体验。
路由 是一种导航方式,类似于我们之前使用的 动态组件。
使用路由,我们可以使用 URL 地址将用户引导到 Vue 应用程序的特定位置。
使用动态组件导航
为了理解 Vue 中的路由,让我们先看看一个使用动态组件在两个组件之间切换的应用程序。
我们可以使用按钮在组件之间切换
示例
FoodItems.vue
:
<template>
<h1>Food!</h1>
<p>I like most types of food.</p>
</template>
AnimalCollection.vue
:
<template>
<h1>Animals!</h1>
<p>I want to learn about at least one new animal every year.</p>
</template>
App.vue
:
<template>
<p>Choose what part of this page you want to see:</p>
<button @click="activeComp = 'animal-collection'">Animals</button>
<button @click="activeComp = 'food-items'">Food</button><br>
<div>
<component :is="activeComp"></component>
</div>
</template>
<script>
export default {
data() {
return {
activeComp: ''
}
}
}
</script>
<style scoped>
button {
padding: 5px;
margin: 10px;
}
div {
border: dashed black 1px;
padding: 20px;
margin: 10px;
display: inline-block;
}
</style>
运行示例 »
从动态组件到路由
我们使用 Vue 构建 SPA(单页应用程序),这意味着我们的应用程序只包含一个 *.html 文件。这意味着我们无法将用户引导到其他 *.html 文件来显示页面上的不同内容。
在上面的示例中,我们可以导航到页面上的不同内容,但我们无法向其他人提供页面的地址,以便他们直接进入有关食物的部分,但使用路由我们可以做到。
通过适当设置路由,如果你使用 URL 地址的扩展打开 Vue 应用程序,例如 "/food-items",你将直接进入包含食物内容的部分。
安装 Vue Router 库
要在你的机器上使用 Vue 中的路由,请使用终端在你的项目文件夹中安装 Vue Router 库
npm install vue-router@4
更新 main.js
为了使用路由,我们必须创建一个路由器,我们在 main.js 文件中执行此操作。
main.js
:
import { createApp } from 'vue'
import { createRouter, createWebHistory } from 'vue-router'
import App from './App.vue'
import FoodItems from './components/FoodItems.vue'
import AnimalCollection from './components/AnimalCollection.vue'
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/animals', component: AnimalCollection },
{ path: '/food', component: FoodItems },
]
});
const app = createApp(App)
app.use(router);
app.component('food-items', FoodItems);
app.component('animal-collection', AnimalCollection);
app.mount('#app')
添加了第 2 行、第 8-14 行和第 18 行以添加路由功能。
删除了第 19-20 行,因为组件已经通过第 11-12 行中的路由包含在内。
我们现在创建了一个路由器,例如,如果在原始 URL 地址的末尾添加 "/animals",它可以打开 'AnimalCollection' 组件,但它在下一节添加 <router-view>
组件之前不会起作用。路由器还跟踪网页历史记录,以便你可以使用通常位于网页浏览器左上角的 URL 旁边的箭头在历史记录中前进和后退。
使用 <router-view> 组件
为了使用新的路由器更改页面上的内容,我们需要删除上一个示例中的动态组件,并使用 <router-view>
组件代替。
App.vue
:
<template>
<p>Choose what part of this page you want to see:</p>
<button @click="activeComp = 'animal-collection'">Animals</button>
<button @click="activeComp = 'food-items'">Food</button><br>
<div>
<router-view></router-view>
<component :is="activeComp"></component>
</div>
</template>
如果你在你的计算机上完成了上述更改,你可以在浏览器中将 "/food" 添加到你的项目页面的 URL 地址,页面应该更新以显示食物内容,如下所示
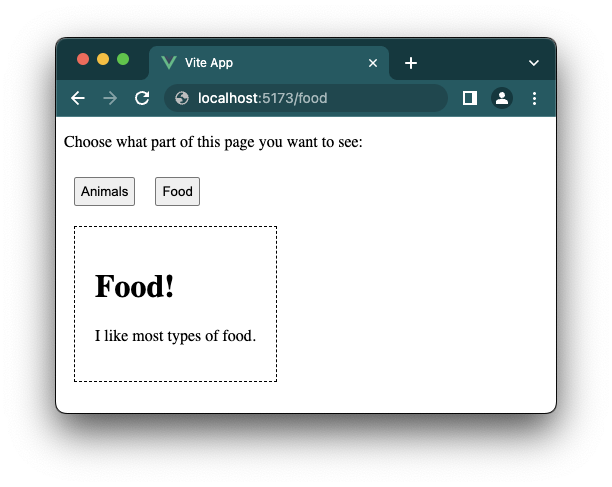
使用 <router-link> 组件
我们可以用 <router-link>
组件替换按钮,因为这更适合路由器。
我们不再需要 'activeComp' 数据属性,因此可以删除它,实际上可以删除整个 <script>
标签,因为它为空。
App.vue
:
<template>
<p>Choose what part of this page you want to see:</p>
<router-link to="/animals">Animals</router-link>
<router-link to="/food">Food</router-link><br>
<div>
<router-view></router-view>
</div>
</template>
<script></script>
为 <router-link> 组件设置样式
<router-link>
组件被渲染为 <a>
标签。如果我们在浏览器中右键单击元素并检查它,我们可以看到这一点
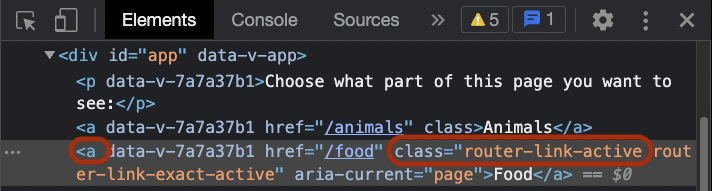
正如你在上面的屏幕截图中看到的,Vue 也跟踪哪个组件处于活动状态,并为活动 <router-link>
组件(现在被渲染为 <a>
标签)提供 'router-link-active' 类。
我们可以使用上面的信息来设置样式以突出显示哪个 <router-link>
组件处于活动状态
示例
App.vue
:
<template>
<p>Choose what part of this page you want to see:</p>
<router-link to="/animals">Animals</router-link>
<router-link to="/food">Food</router-link><br>
<div>
<router-view></router-view>
</div>
</template>
<style scoped>
a {
display: inline-block;
background-color: black;
border: solid 1px black;
color: white;
padding: 5px;
margin: 10px;
}
a:hover,
a.router-link-active {
background-color: rgb(110, 79, 13);
}
div {
border: dashed black 1px;
padding: 20px;
margin: 10px;
display: inline-block;
}
</style>
运行示例 »
注意:在上面的示例中,URL 地址没有更新,但如果你在自己的机器上执行此操作,URL 地址将更新。上面的示例即使 URL 地址没有更新也能正常工作,因为路由是由 Vue 中的路由器在内部处理的。